Java Shot #5: The Hidden Pitfall of Using toString() in Enums
Let's understand the difference between toString() and name() while working with Enum class in Java
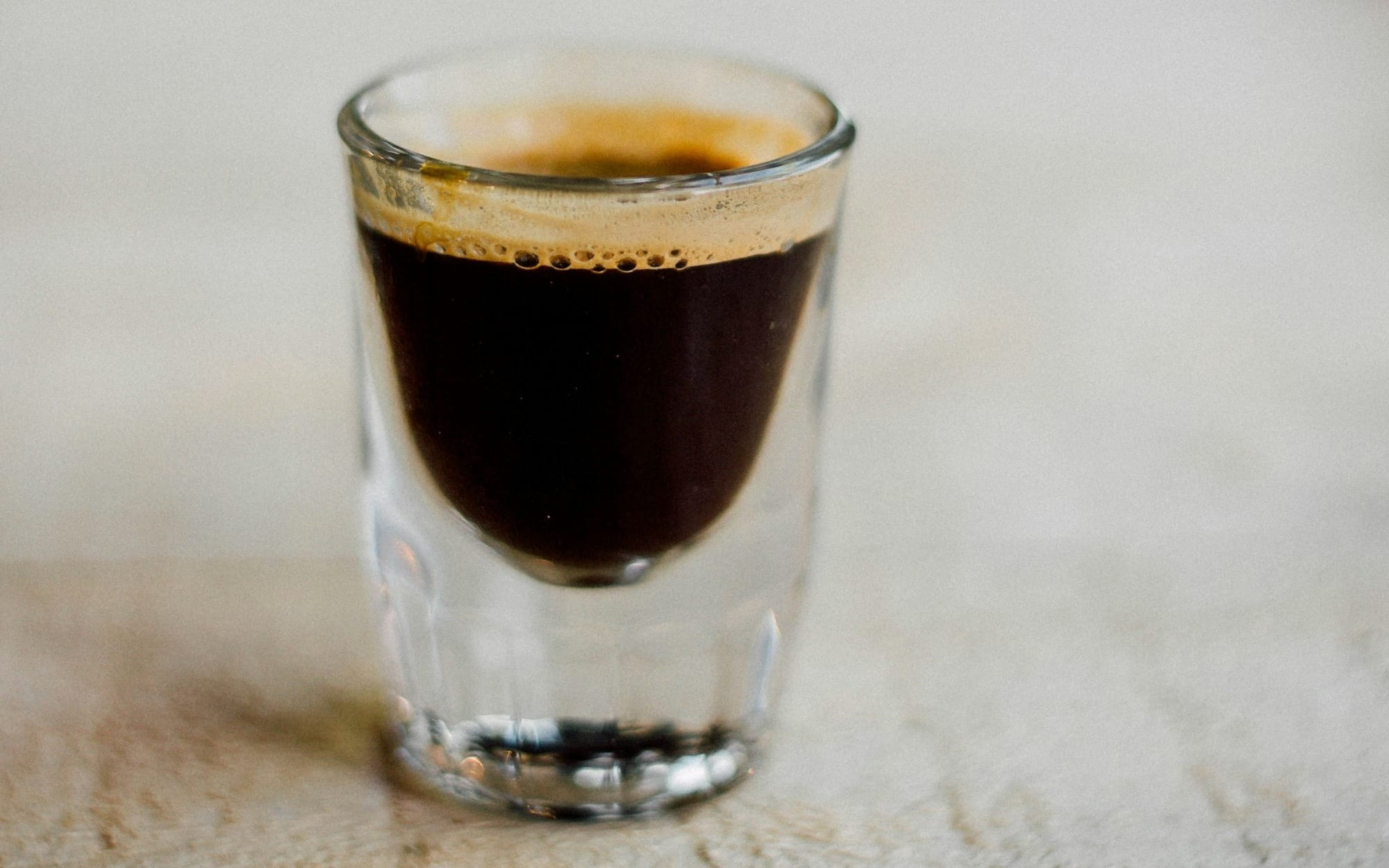
It's a common practice in code to convert String to Enum and vice-versa.
String to Enum Conversion
String to Enum is pretty straight-forward and there's only one way to do it - which is using valueOf()
method from the Enum class.
Let's take an example:
// DayOfWeek.java
public enum DayOfWeek {
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY,
SUNDAY;
}
// EnumExample.java
public class EnumExample {
public static void main(String[] args) {
String dayString = "WEDNESDAY";
try {
DayOfWeek day = DayOfWeek.valueOf(dayString.toUpperCase());
System.out.println("Converted string to enum: " + day);
} catch (IllegalArgumentException e) {
System.out.println("Invalid day string: " + dayString);
}
}
}
For this enum class, valueOf()
works like a charm. If someone's telling you there's a better way of doing it, it could be over-engineering (If not, comment below, let's discuss)
Enum to String Conversion
Let's try Enum to String Conversion.
- By overriding
toString()
method.
// DayOfWeek.java
@ToString // assuming that toString() is overridden with the help of this annotation.
public enum DayOfWeek {
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY,
SUNDAY;
}
// EnumExample.java
public class EnumExample {
public static void main(String[] args) {
DayOfWeek monday = DayOfWeek.MONDAY;
System.out.println(monday.toString()); // MONDAY
}
}
- By using
name()
method.
// DayOfWeek.java
@ToString // assuming that toString() is generated with the help of this annotation.
public enum DayOfWeek {
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY,
SUNDAY;
}
// EnumExample.java
public class EnumExample {
public static void main(String[] args) {
DayOfWeek monday = DayOfWeek.MONDAY;
System.out.println(monday.name()); // outputs: MONDAY
}
}
The output of the following two approaches seems to be the same and I have come across code where toString()
also used for converting an enum into a String.
This is not wrong in the above context but let's take another example where using toString()
is incorrect.
public enum DayOfWeek {
MONDAY(1, "Mon"),
TUESDAY(2, "Tue"),
WEDNESDAY(3, "Wed"),
THURSDAY(4, "Thu"),
FRIDAY(5, "Fri"),
SATURDAY(6, "Sat"),
SUNDAY(7, "Sun");
private final int dayNumber;
private final String shortName;
DayOfWeek(int dayNumber, String shortName) {
this.dayNumber = dayNumber;
this.shortName = shortName;
}
public int getDayNumber() {
return dayNumber;
}
public String getShortName() {
return shortName;
}
public static DayOfWeek fromNumber(int number) {
for (DayOfWeek day : values()) {
if (day.dayNumber == number) {
return day;
}
}
throw new IllegalArgumentException("Invalid day number: " + number);
}
// How the tool-generated toString() code looks like.
// For Monday, it would output "MONDAY (1, Mon)" and not "MONDAY".
// This is invalid enum and hence it's recommended to use name() to get
// the exact enum value.
@Override
public String toString() {
return name() + " (" + dayNumber + ", " + shortName + ")";
}
}
Read the comments above toString() method for more info.
If you observe the toString()
method implementation, you will understand why we should avoid using it for the conversion of an enum into a String.
This is a silly mistake but you might end up missing it. I learnt it the hard way ;)